带交叉验证的ROC曲线¶
这个案例展示了使用交叉验证来评估分类器输出质量的接收器操作特征(ROC)曲线。
ROC曲线通常在Y轴上具有真正率(true positive rate),在X轴上具有假正率(false positive rate)。这意味着该图的左上角是“理想”点-误报率为零,而正误报率为1。这不是很现实,但这确实意味着,通常来说,曲线下的区域(AUC面积)越大越好。
ROC曲线的“陡峭程度”也很重要,因为理想的是最大程度地提高真正率,同时最小化假正率。
此示例显示了通过K折交叉验证创建的不同数据集的ROC曲线。取所有这些曲线,可以计算出曲线下的平均面积,并在训练集分为不同子集时查看曲线的方差。这大致显示了分类数据的输出如何受到训练数据变化的影响,以及由K折交叉验证产生的拆分之间的差异如何。
注意:同事也需要查看这些链接:sklearn.metrics.roc_auc_score
,
sklearn.model_selection.cross_val_score
,ROC曲线
输出:
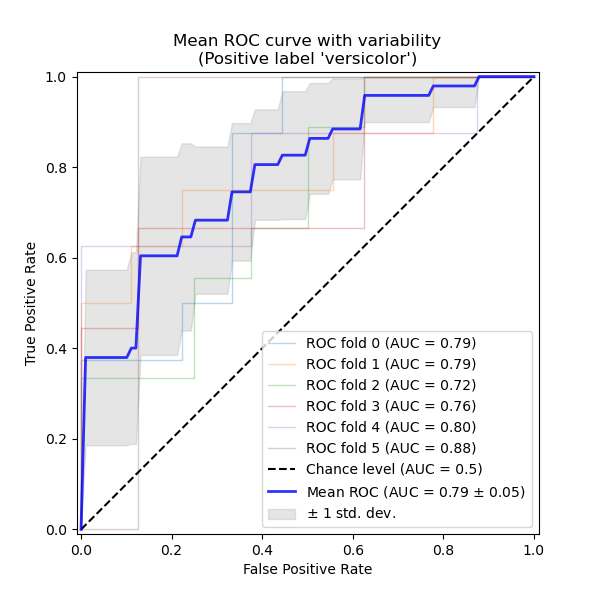
输入:
print(__doc__)
import numpy as np
import matplotlib.pyplot as plt
from sklearn import svm, datasets
from sklearn.metrics import auc
from sklearn.metrics import plot_roc_curve
from sklearn.model_selection import StratifiedKFold
# #############################################################################
# 获取数据
# 导入待处理的数据
iris = datasets.load_iris()
X = iris.data
y = iris.target
X, y = X[y != 2], y[y != 2]
n_samples, n_features = X.shape
# 增加一些噪音特征
random_state = np.random.RandomState(0)
X = np.c_[X, random_state.randn(n_samples, 200 * n_features)]
# #############################################################################
# 分类,并使用ROC曲线分析结果
# 使用交叉验证运行分类器并绘制ROC曲线
cv = StratifiedKFold(n_splits=6)
classifier = svm.SVC(kernel='linear', probability=True,
random_state=random_state)
tprs = []
aucs = []
mean_fpr = np.linspace(0, 1, 100)
fig, ax = plt.subplots()
for i, (train, test) in enumerate(cv.split(X, y)):
classifier.fit(X[train], y[train])
viz = plot_roc_curve(classifier, X[test], y[test],
name='ROC fold {}'.format(i),
alpha=0.3, lw=1, ax=ax)
interp_tpr = np.interp(mean_fpr, viz.fpr, viz.tpr)
interp_tpr[0] = 0.0
tprs.append(interp_tpr)
aucs.append(viz.roc_auc)
ax.plot([0, 1], [0, 1], linestyle='--', lw=2, color='r',
label='Chance', alpha=.8)
mean_tpr = np.mean(tprs, axis=0)
mean_tpr[-1] = 1.0
mean_auc = auc(mean_fpr, mean_tpr)
std_auc = np.std(aucs)
ax.plot(mean_fpr, mean_tpr, color='b',
label=r'Mean ROC (AUC = %0.2f $\pm$ %0.2f)' % (mean_auc, std_auc),
lw=2, alpha=.8)
std_tpr = np.std(tprs, axis=0)
tprs_upper = np.minimum(mean_tpr + std_tpr, 1)
tprs_lower = np.maximum(mean_tpr - std_tpr, 0)
ax.fill_between(mean_fpr, tprs_lower, tprs_upper, color='grey', alpha=.2,
label=r'$\pm$ 1 std. dev.')
ax.set(xlim=[-0.05, 1.05], ylim=[-0.05, 1.05],
title="Receiver operating characteristic example")
ax.legend(loc="lower right")
plt.show()
脚本的总运行时间:(0分钟0.403秒)